With powerful features like server-side rendering (SSR), static site generation (SSG), and automatic code splitting, Next.js has become one of the most popular frameworks for making React apps. Before you start developing with Next.js, you must make sure you have certain skills to use the framework.
Let's look at the most important skills that every Next.js coder should have.
1. Strong Understanding of React
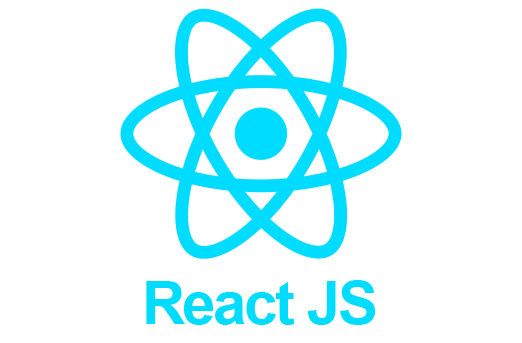
Next.js is essentially a React framework, so being proficient in React is a must. Important concepts include:
- Components: Understanding functional components and hooks is crucial as it forms the backbone of how Next.js pages are structured.
- JSX Syntax: React’s unique syntax for rendering HTML in JavaScript code will be central to your Next.js development.
- Hooks (useState, useEffect, etc.): Hooks are essential for handling state and side effects in functional components.
- State and Props: These concepts are at the core of React component interactions, and managing them correctly is key to a smooth development experience.
In Next.js, pages are often React components, so mastering React ensures you're well-prepared for Next.js development.
2. Familiarity with Server-Side Rendering (SSR)
Next.js excels in server-side rendering, which can improve SEO and load times by rendering pages on the server before sending them to the client. To make the most of SSR, you should understand:
- getServerSideProps: This Next.js function enables you to fetch data on each request and render it on the server.
- SSR vs. Client-Side Rendering (CSR): Knowing the difference and when to use each can optimize your app’s performance.
- SEO benefits: Since SSR ensures your page content is available to search engines, understanding the SEO advantages is important for creating performant web apps.
3. Static Site Generation (SSG)
Next.js also supports static site generation, where pages are pre-rendered at build time. This improves performance and scalability. You’ll need to understand:
- getStaticProps: Similar to SSR but used for building static pages during the build process.
- Incremental Static Regeneration (ISR): Next.js allows you to update static pages after they’ve been generated. Understanding how to regenerate specific pages based on user interaction or updates is crucial for dynamic apps.
- Use cases for SSG: Knowing when to use static generation instead of SSR or CSR will help you optimize page load times and scalability.
4. API Routes and Integration
API routes are an integral part of Next.js, allowing you to create your own API endpoints without needing a separate server. To make the most of this feature, you should:
- Understand API routes: Learn how to create serverless functions using API routes that handle requests and send responses.
- Integration with third-party APIs: Whether you’re fetching data from external services or interacting with other APIs, understanding how to work with both RESTful and GraphQL APIs is essential.
- Data fetching: Knowing how to fetch data from external APIs using getStaticProps, getServerSideProps, and client-side data fetching is key to creating dynamic apps.
5. Routing in Next.js
Routing in Next.js is quite different from traditional React apps, as it is file-system-based. Every file in the pages directory automatically becomes a route. To navigate and manage routes effectively, you should be familiar with:
- Dynamic Routing: Learn how to create dynamic routes with file names in square brackets (e.g., [id].js).
- Nested Routing: Understanding how to structure nested directories within the pages folder for more complex apps.
- API Route Routing: Knowing how to create custom API routes based on the file structure is key to leveraging Next.js as a full-stack framework.
6. CSS-in-JS and Styling Solutions
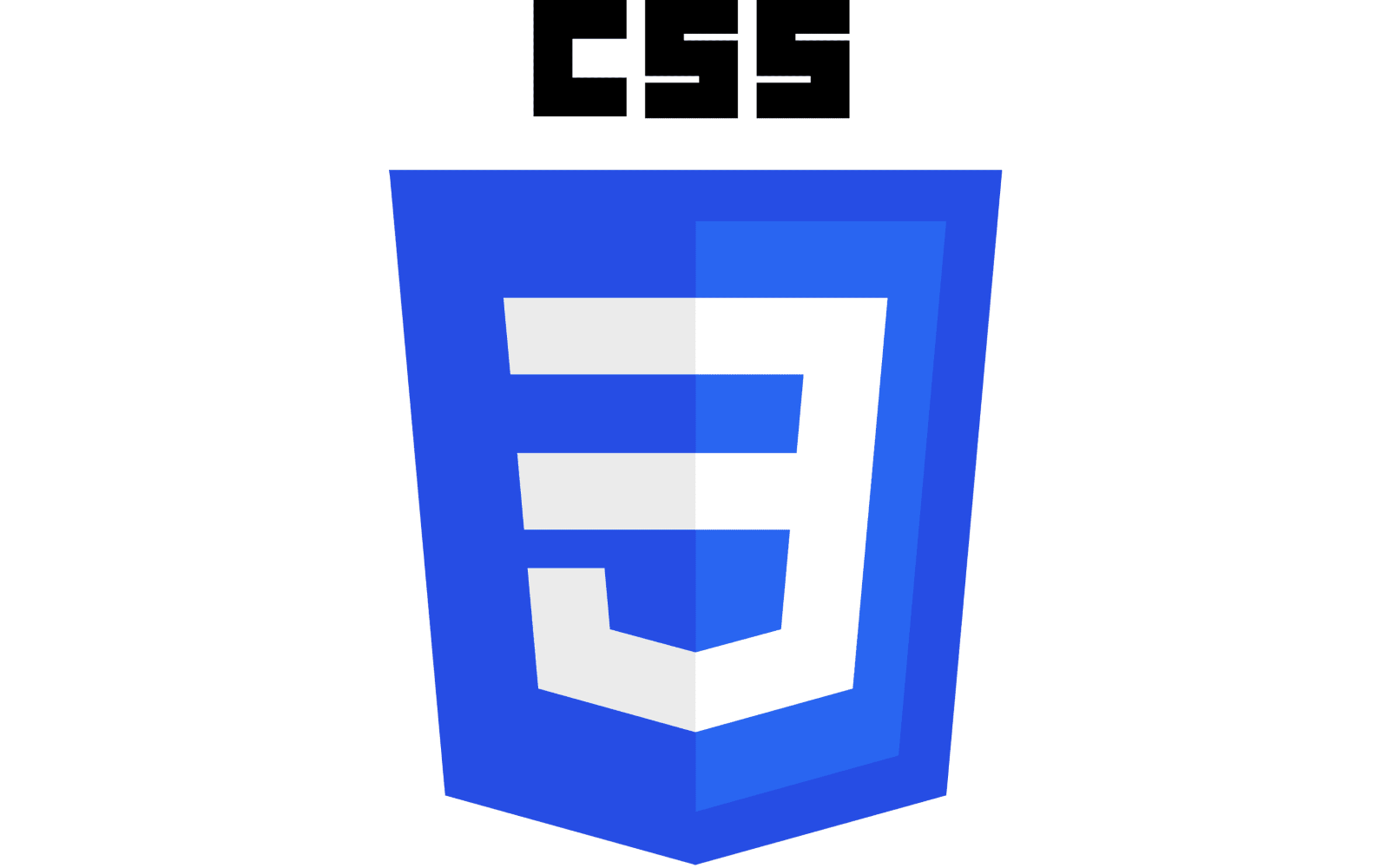
Next.js supports various styling methods, but it excels at CSS-in-JS solutions. You can style your components using different approaches like:
- Styled Components or Emotion: These libraries let you write traditional CSS syntax within JavaScript files, scoped to individual components.
- CSS Modules: Next.js supports CSS modules natively, providing scoped and modular CSS for specific components without global scope pollution.
- Global Styles: In case you need to apply global styles, understanding how to use the globals.css file or apply global styles within your component is essential.
7. TypeScript (Optional but Recommended)

Although optional, TypeScript is gaining popularity in the Next.js ecosystem. TypeScript adds static typing to JavaScript, helping you catch errors early in development. You should learn:
- Setting up TypeScript in Next.js: The framework has built-in TypeScript support, making it easy to get started.
- Type Annotations: Understanding how to annotate props, state, and API functions can make your code more reliable.
- Error handling: TypeScript helps you handle unexpected behavior by enforcing type checks, which results in more stable apps.
8. Deployment and Optimization
Finally, you need to know how to deploy your Next.js apps efficiently. Next.js is often deployed to platforms like Vercel, but you can also use traditional platforms like AWS or DigitalOcean. Essential skills include:
- Deployment workflows: Understanding how to deploy using CI/CD pipelines ensures smoother updates and scalability.
- Performance Optimization: Learn how to optimize bundle sizes, use lazy loading, and leverage Next.js features like automatic image optimization and static exports.
- Serverless functions: Know how to deploy serverless functions, particularly when using API routes in a production environment.
Conclusion
This is a strong framework called Next.js that can handle both static and dynamic web apps. It combines the best of React and server-side features. If you want to be good at Next.js programming, you need to know a lot about JavaScript, React, and ideas like SSR, SSG, API integration, routing, and styling. If you learn these skills, it will be easy to make apps that are fast, scalable, and good for SEO.
With these essential skills, you’ll be well-prepared to take on Next.js projects and fully leverage the framework’s capabilities.
Sign up at Pro5 and get connected with us in kickstarting your career in Next.js development!
Visit Pro5 today!