Angular is one of the most popular frameworks for building dynamic, scalable web applications. Whether you're a beginner or looking to level up your front-end skills, mastering Angular requires a solid foundation in several key areas. Here are the essential skills you need to become a proficient Angular developer.
1. Mastering TypeScript
Angular is written in TypeScript, a statically-typed superset of JavaScript. Learning TypeScript is fundamental to Angular development because it introduces important features like types, interfaces, and decorators, which help in writing safer and more maintainable code. TypeScript's static typing system catches errors at compile-time, meaning you can avoid common runtime errors and improve overall code quality. This is particularly helpful in larger applications where code organization and debugging can become more complex. Learning TypeScript will not only improve your Angular skills but also your overall JavaScript development, as it's increasingly used in modern JavaScript frameworks.
Example: If you're building a large-scale application, TypeScript helps prevent common JavaScript errors by enforcing types. This can save hours of debugging down the line.
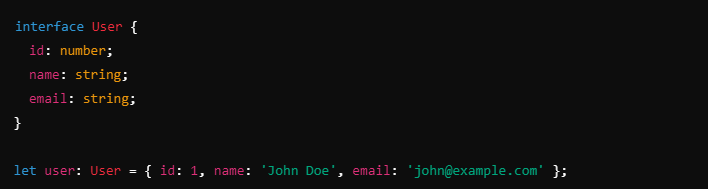
2. Understanding Angular Modules and Components
Components are the building blocks of any Angular application, and understanding their architecture is key to structuring your app. A component in Angular consists of three parts: a TypeScript class, an HTML template, and CSS styles. Each component is a self-contained unit of the user interface, responsible for displaying data and handling user interactions. Modules in Angular are used to group together related components, services, and other code. Understanding how modules and components work together is vital to writing modular, reusable, and maintainable code. Proper organization using modules helps manage application complexity, especially as the codebase grows.
Example: Each Angular app has a root module (AppModule) that bootstraps your application, and components such as AppComponent manage different parts of your UI.
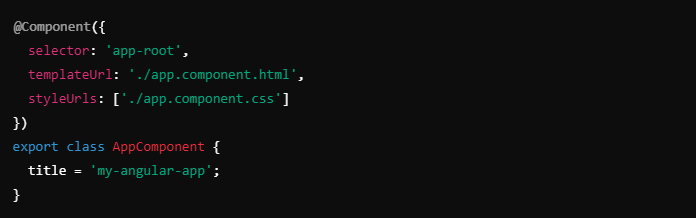
3. Working with Angular CLI
The Angular Command Line Interface (CLI) is a powerful tool that simplifies various development tasks. From creating new components to building the project for production, the CLI handles much of the grunt work. It allows developers to generate new services, directives, and modules with a single command, saving valuable development time. Additionally, the CLI includes options for building and optimizing your application for deployment, running tests, and creating test files. Familiarizing yourself with the Angular CLI not only boosts productivity but also ensures consistency across your codebase, as it follows Angular’s best practices.
Tip: Use the ng generate command to quickly scaffold components or services.

4. Reactive Programming with RxJS
One of the most powerful concepts in Angular is reactive programming, which is handled by RxJS (Reactive Extensions for JavaScript). RxJS allows you to work with asynchronous data streams, which is essential for handling operations like HTTP requests, WebSocket connections, and user input. Reactive programming makes managing complex event-driven interactions in an Angular app more efficient and manageable. Instead of handling asynchronous operations manually, RxJS allows you to use Observables, which can be combined, filtered, or transformed using operators. Learning RxJS is a must for anyone wanting to master Angular, as it's deeply integrated into the framework, especially when dealing with forms, HTTP requests, and state management.
Example: Use HttpClient in Angular to fetch data from an API and handle it as an Observable.

5. Creating and Handling Forms
Forms are integral to almost every web application, and Angular provides two approaches for handling them: template-driven forms and reactive forms. Template-driven forms are simpler and more intuitive for small, straightforward forms, whereas reactive forms offer more control, flexibility, and scalability for complex forms. Reactive forms are especially useful when you need dynamic form creation or complex form validation. Mastering Angular forms also involves understanding how to manage form state, validation, and user input. Choosing the right form approach for your project is essential for creating efficient, user-friendly web applications.
Example: Reactive forms offer more control over the form’s data model and are perfect for dynamic form creation.

6. Routing and Navigation
Angular's Router module is essential for building single-page applications (SPAs) that provide a seamless user experience by navigating between different views or pages without reloading the entire page. Routing in Angular allows you to define routes that correspond to different components and pass data between them. Additionally, you can implement route guards to protect certain routes, lazy-load modules to improve performance, and even resolve route data before the view is rendered. Mastering Angular routing is critical to building responsive, smooth web applications that feel like native desktop or mobile apps.
Example: Define routes for your application in app-routing.module.ts and use routerLink to navigate between views.

7. State Management
As your application grows in size, managing state (the data that drives your app) becomes increasingly challenging. State management libraries like NgRx implement the Redux pattern to manage the global state of your application. NgRx helps you ensure that the state is predictable and that components receive data consistently. Understanding state management patterns is crucial, especially in applications with complex workflows, many components, or heavy data interaction. Learning how to manage application state with tools like NgRx will help you create scalable, maintainable applications.
Example: Use NgRx to manage the state of your application, ensuring that your data remains consistent across components.

8. Testing in Angular
Testing is an essential part of developing any software application, and Angular provides built-in tools for both unit testing and end-to-end (E2E) testing. Jasmine is used for unit tests, and Karma is used as a test runner to automate them. For E2E testing, Angular includes Protractor, which allows you to simulate user interactions and ensure the entire application works as expected. Writing tests for your components, services, and modules ensures that your app is reliable and less prone to bugs. Proper testing practices also lead to easier maintenance and refactoring down the line.
Example: Write a basic test for an Angular component using Jasmine.

Conclusion
Mastering Angular requires a diverse set of skills, from TypeScript fundamentals to advanced topics like RxJS and state management. By focusing on these essential areas, you'll be well-equipped to build robust and scalable Angular applications.
Whether you're developing a small single-page app or a large enterprise solution, these skills will set you up for success. Each of these skills complements the others, forming the foundation of a strong Angular developer's toolkit.