Are you a beginner looking to break into the world of programming?
Perhaps you've heard of C++, one of the most popular and versatile programming languages out there. Despite its reputation for being complex and intimidating, C++ can be a great language to start with as a beginner.
In this beginner's guide for C++ developers, we'll take a deep dive into the following:
- What is C++?
- Setting up the Development Environment
- Basic Syntax
- Functions
- Arrays and Strings
- Pointers
- Object-Oriented Programming
- Input and Output in C++
- Standard Libraries of C++
By the end of this guide, you'll have a solid understanding of the C++ language and be well on your way to creating powerful applications that can run on a variety of platforms. So let's get started on this exciting journey into the world of C++ programming!
What is C++?
C++ is a powerful and efficient programming language that is widely used for developing software applications, system software, operating systems, embedded systems, games, and more. It is an object-oriented programming language, which means it is based on the concepts of objects and classes that enable modular programming, code reuse, and abstraction.
C++ is an intermediate-level language, which means it is more complex than high-level languages like Python and easier to learn than low-level languages like Assembly.
It is deemed an important language because of its versatility, performance, and popularity. C++ is used by many large tech companies like Google, Microsoft, Amazon, and Adobe. It is also the language of choice for developing high-performance software like gaming engines, financial trading systems, and scientific simulations.
Brief History of C++
C++ was developed by Bjarne Stroustrup in 1983 at Bell Labs in Murray Hill, New Jersey. Stroustrup wanted to create an extension of the C language that could support object-oriented programming concepts like classes and inheritance. Stroustrup named the language "C with Classes" and later renamed it to "C++" (pronounced "see plus plus").
The first official release of C++ was in 1985, and it was based on the C programming language. C++ quickly gained popularity among programmers because of its object-oriented features, type safety, and performance. In 1998, the International Organization for Standardization (ISO) standardized C++, which resulted in the release of the C++98 standard.
Setting up the Development Environment
C++ is a powerful and widely-used programming language that requires a proper development environment to be set up before starting any programming project. In this blog, we will walk you through the process of setting up a C++ development environment by installing an IDE and a compiler.
1. Installing an IDE (Integrated Development Environment)
An IDE is a software application that provides a comprehensive development environment for programmers to write, test, and debug code. There are many IDEs available for C++ development, but we recommend using Visual Studio Code (VS Code) as it is a lightweight and powerful IDE that is free and open-source.
To install VS Code for C++ development, follow these steps:
- Download and install VS Code from the official website.
- Open VS Code and click on the "Extensions" icon on the left-hand side of the screen.
- Search for the "C++" extension and install it.
- Restart VS Code to activate the C++ extension.
Now, your development environment is ready to start coding in C++ using VS Code.
2. Installing a Compiler
A compiler is a software tool that translates source code written in a programming language into machine code that can be executed by a computer. For C++ development, the most popular compiler is the GNU Compiler Collection (GCC), which is a free and open-source compiler that supports many platforms and architectures.
To install GCC for C++ development, follow these steps:
- Download and install MinGW-w64, which is a free and open-source compiler for Windows that includes GCC.
- During the installation process, select the "C++" component to install the necessary C++ libraries and headers.
- Add the path to the bin folder of the MinGW-w64 installation directory to the system PATH environment variable.
Now, your development environment is ready to compile and execute C++ programs using the GCC compiler.
Basic Syntax in C++
The basic syntax of C++ includes the structure of a C++ program, variables and data types, operators, and control flow statements.
1. Structure of a C++ program
The structure of a C++ program consists of a series of statements that are executed sequentially. Each statement can be a declaration, expression, or control flow statement. A typical C++ program starts with a header file that contains preprocessor directives, followed by the main() function that contains the program logic.
The basic structure of a C++ program is as follows:
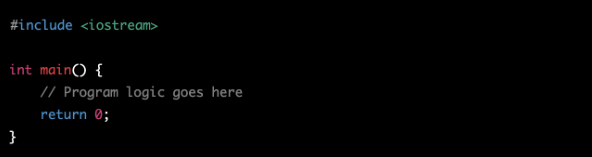
2. Variables and Data Types
Variables in C++ are used to store data and can be of different types, such as integer, float, double, boolean, and more. To declare a variable in C++, you need to specify the data type followed by the variable name.
For example, to declare an integer variable called num, you can use the following statement:

You can also assign a value to the variable at the time of declaration, like this:

3. Operators
Operators in C++ are used to perform operations on variables and data. There are different types of operators in C++, such as arithmetic operators, assignment operators, comparison operators, logical operators, and more.
For example, the arithmetic operators in C++ include ‘+’, ‘-’, ‘*’, ‘/’, and ‘%’, which are used to perform addition, subtraction, multiplication, division, and modulus operations, respectively.
4. Control Flow Statements
Control flow statements in C++ are used to control the flow of execution in a program based on certain conditions. There are different types of control flow statements in C++, such as if-else statements, switch statements, while loops, for loops, and more.
For example, an if-else statement in C++ is used to execute a certain block of code if a certain condition is true and a different block of code if the condition is false, like this:

By following the guidelines outlined above, you can start writing C++ programs and explore its many features and capabilities.
Functions in C++
1. Definition and Syntax of Functions
Functions in C++ are blocks of code that perform a specific task. They can be called from other parts of the program to execute the code within them. Functions in C++ can be defined in two parts: the declaration and the definition.
The declaration of a function specifies its name, return type, and arguments. The definition of a function specifies the actual code to be executed when the function is called.
Here's an example of a function declaration and definition:
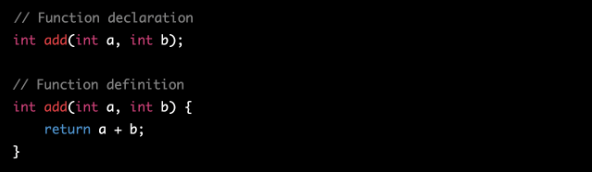
2. Return Types and Arguments
In C++, functions can return a value or not, depending on the needs of the program. The return type of a function is specified in the function declaration, and it specifies the type of value the function returns. If a function doesn't return a value, its return type is void.
Functions in C++ can also have arguments, which are inputs to the function. Arguments are specified in the function declaration, and their types must match the types specified in the function definition.
Here's an example of a function with a return type and arguments:
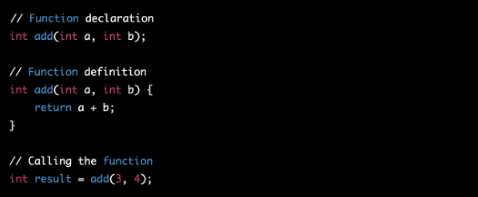
In this example, the ‘add()’ function takes two integer arguments and returns their sum. The function is then called with the values 3 and 4, and the result is assigned to the variable ‘result’.
3. Overloading Functions
C++ allows you to define multiple functions with the same name but different parameter lists. This is known as function overloading. When a function is overloaded, the correct version of the function is called based on the arguments passed to it.
Here's an example of function overloading:

In this example, two versions of the add() function are defined: one that takes two integer arguments and returns an integer, and another that takes two double arguments and returns a double. The correct version of the function is called based on the arguments passed to it.
Writing efficient and flexible programs in C++ is possible when you have a grasp of defining functions, specifying their return types and arguments, and overloading them.
Arrays and Strings in C++
Arrays and strings are both used to store collections of data in C++. An array is a collection of elements of the same data type, while a string is a collection of characters.
Here's an example of how to define an array and a string in C++:
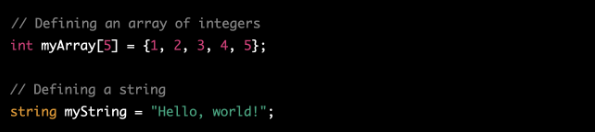
You can access individual elements of an array using their index. The index of an array element starts at 0 for the first element and increases by 1 for each subsequent element.
Here's an example of how to access an element of an array:

In this example, the variable ‘myElement’ is assigned the value of the third element of the ‘myArray’ array, which is 3.
C++ also provides several built-in functions for manipulating strings. These functions can be used to concatenate, compare, and modify strings.
Here are some examples of string manipulation functions:

In C++, powerful and flexible programs can be written by gaining a good understanding of accessing array elements, defining arrays, and utilizing string manipulation functions.
Pointers in C++
1. Definition and Syntax in Pointers
A pointer is a variable that stores the memory address of another variable. In C++, pointers are used to manipulate data directly in memory, and are essential for tasks such as dynamic memory allocation and passing arguments by reference.
Here's an example of how to define and use a pointer in C++:

In this example, the ‘int*’ syntax indicates that ‘myPtr’ is a pointer to an integer. The ‘&’ operator is used to get the memory address of the ‘myVar’ variable.
2. Pointer Arithmetic
In C++, you can perform arithmetic operations on pointers to move them to different memory locations. This can be useful when working with arrays or when dynamically allocating memory.
Here are some examples of pointer arithmetic:

In this example, the ‘myPtr++’ operation moves the pointer to the next element of the array. The ‘*myPtr’ syntax is used to dereference the pointer and get the value stored at that memory location.
3. Dynamic Memory Allocation
In C++, you can allocate memory dynamically at runtime using the new operator. This allows you to create arrays or objects whose size is not known at compile time.
Here's an example of how to allocate and use memory dynamically:

In this example, the ‘new’ operator is used to allocate an array of 5 integers. The ‘[]’ syntax indicates that this is an array allocation. The ‘delete[]’ operator is used to free the memory allocated by ‘new’.
By understanding how to define and use pointers, perform pointer arithmetic, and allocate memory dynamically, you can write powerful and flexible programs in C++.
Object-Oriented Programming in C++
1. Classes and Objects
Object-oriented programming (OOP) is a programming paradigm that emphasizes the use of objects, which are instances of classes, to represent and manipulate data. In C++, classes are defined using the class keyword, and objects are created using the new operator.
Here's an example of how to define a class and create an object in C++:

In this example, the ‘Rectangle’ class has two public member variables, ‘width’ and ‘height.’ The new operator is used to create a new Rectangle object, which is then accessed using the -> operator.
2. Encapsulation, Inheritance, and Polymorphism
Encapsulation, inheritance, and polymorphism are three key concepts of OOP that are supported by C++.
Encapsulation is the idea of hiding the implementation details of a class from the user, and providing a simple interface for accessing the class's functionality. In C++, this is often achieved using private member variables and public member functions.
Inheritance is the idea of creating new classes that inherit the properties and methods of existing classes. In C++, inheritance is achieved using the ‘public’, ‘private’, and ‘protected’ access modifiers.
Polymorphism is the idea of creating objects that can take on multiple forms. In C++, polymorphism is achieved using virtual functions and abstract classes.
Here's an example of how to use encapsulation, inheritance, and polymorphism in C++:
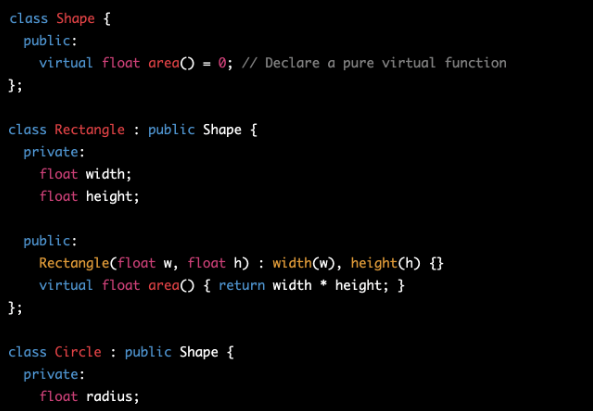

In this example, the ‘Shape’ class is an abstract class with a pure virtual function ‘area()’. The ‘Rectangle’ and ‘Circle‘ classes inherit from ‘Shape’, and implement the ‘area()’ function differently. The ‘myShape’ pointer is used to point to different objects at runtime, demonstrating polymorphism.
Writing powerful and flexible programs in C++ is possible when you have a good understanding of defining classes and objects, as well as utilizing concepts such as encapsulation, inheritance, and polymorphism.
Input and Output in C++
1. Console Input and Output
C++ provides a variety of ways to perform console input and output operations. The most common ones include ‘cout’, ‘cin’, and ‘cerr’.
‘cout' is used to output data to the console, while ‘cin’ is used to read data from the console. Here's an example of how to use ‘cout’ and ‘cin’ in C++:
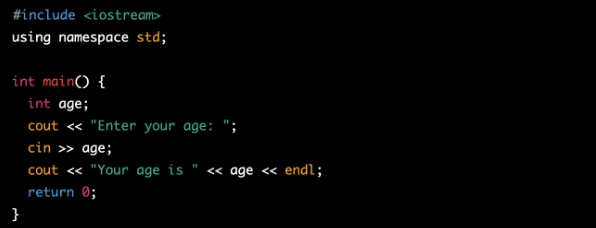
In this example, the ‘<<’ operator is used with ‘cout’ to output a prompt to the console, while the ‘>>’ operator is used with ‘cin’ to read the user's input. The ‘endl’ manipulator is used to output a newline character.
‘cerr’ is used to output error messages to the console. It's similar to ‘cout’, but is typically used for debugging purposes. Here's an example of how to use ‘cerr’ in C++:

In this example, the ‘cerr’ statement is used to output an error message if the value of ‘x’ is 0, preventing a potential division by zero error.
2. File Input and Output
C++ also provides a way to read and write data to files. The most common ways to do this are using ‘ofstream’ (output file stream) and ‘ifstream’ (input file stream).
Here's an example of how to use ‘ofstream’ and ‘Ifstream’ in C++:
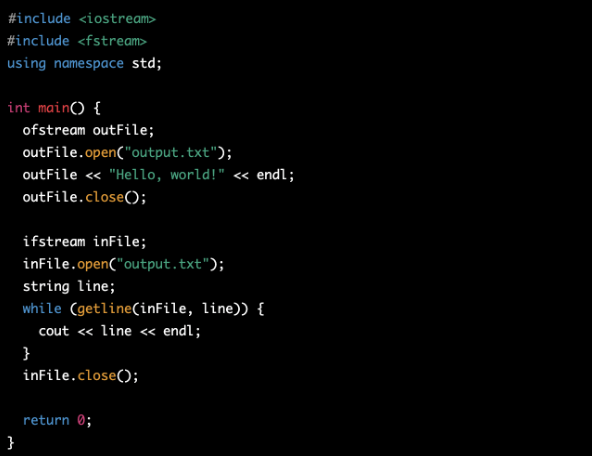
In this example, the ‘ofstream’ object ‘outFile’ is used to open a file named "output.txt" and write the string "Hello, world!" to it. The ‘ifstream’ object ‘inFile’ is then used to open the same file and read the contents into a string variable ‘line’, which is output to the console using ‘cout’. The ‘close()’ method is used to close both the input and output streams.
Gaining knowledge of console input and output, as well as file input and output, can lead to the creation of powerful and flexible programs in C++.
C++ Standard Libraries
One of the key features of C++ is its extensive standard library, which provides a rich set of functions and classes that developers can use to build robust and efficient applications. The C++ standard library includes several components, each of which serves a specific purpose.
1. The Standard Template Library (STL)
The Standard Template Library is perhaps the most well-known component of the C++ standard library. The STL is a collection of classes and algorithms that provide generic solutions for common programming problems. It includes containers, such as arrays, vectors, sets, and maps, as well as algorithms, such as sorting, searching, and manipulating data. The STL also includes iterators, which are used to traverse the elements of a container, and function objects, which provide a way to encapsulate functions as objects.
2. Input/Output (IO) Library
This library provides a set of classes and functions that allow developers to read and write data to and from files, streams, and other input/output devices. The IO library includes classes for reading and writing binary data, formatted text, and character data. It also includes classes for manipulating file pointers and buffering data.
3. Library for working with strings and character data
This library includes functions for converting between different types of character encodings, as well as classes for manipulating strings and individual characters. The string library provides a convenient and efficient way to work with text data in C++.
4. Math Library
The Math Library in C++ provides a set of mathematical functions for working with numbers, and the locale library, which provides a set of classes for working with different locales and character sets. The threading library provides classes and functions for working with threads and concurrency, while the exception library provides a set of classes for handling errors and exceptions in C++.
5. Libraries that provide additional functionality
In addition to these core components, the C++ standard library includes a variety of other libraries and modules that provide additional functionality. For example, the chrono library provides classes and functions for working with time and dates, while the regex library provides support for regular expressions.
Overall, the C++ standard library provides a rich and powerful set of tools that can help developers build efficient and reliable applications. By leveraging the functionality provided by the standard library, developers can save time and effort, while also producing high-quality code.
Conclusion
In this blog, we covered the fundamentals of C++ programming, including its syntax, variables and data types, control flow statements, functions, arrays and strings, pointers, object-oriented programming concepts, input and output operations, and the standard libraries of C++.
Indeed, C++ is a powerful and flexible programming language that can be used to create a wide variety of applications, from small scripts to large-scale software projects. Its syntax can be complex at times, but once you get the hang of it, you'll find that C++ offers unparalleled control and efficiency in your code.
Further Learning and Resources for C++ Beginners
If you're interested in furthering your knowledge of C++, there are many resources available to you. Here are a few that we recommend:
The C++ Programming Language (4th Edition) by Bjarne Stroustrup
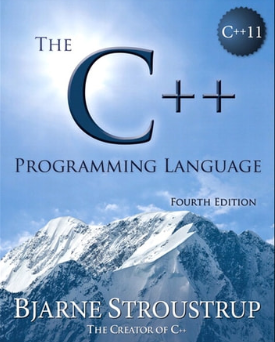
This is the definitive guide to the C++ language, written by the language's creator himself.
C++ Primer (5th Edition) by Stanley B. Lippman, Josée Lajoie, and Barbara E. Moo
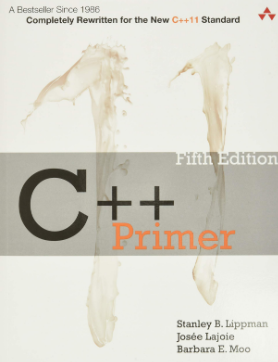
This book provides a comprehensive introduction to C++ programming and covers a wide range of topics, from basic syntax to advanced programming concepts.
learncpp.com

This is a free online resource that provides a comprehensive introduction to C++ programming, with a focus on practical examples and exercises.
Cplusplus.com

This website provides a comprehensive reference guide to the C++ language, including syntax, functions, libraries, and more.
By using these resources and continuing to practice your programming skills, you'll be well on your way to mastering the C++ language and creating powerful applications that can run on a variety of platforms.