C++ is a strong language with a lot of options. However, if you're not careful, this choice can sometimes lead to problems. No matter how much experience you have as a coder, the best way to write strong and efficient C++ code is to avoid making common mistakes.
This blog post will talk about some common mistakes people make when writing C++ code and give you tips on how to avoid them.
1. Uninitialized Variables
Mistake: Using variables before they are initialized is a frequent source of bugs in C++. Unlike some languages that automatically initialize variables, C++ does not. Accessing an uninitialized variable leads to undefined behavior, which can manifest as crashes or incorrect results.

How to Avoid: Always initialize your variables when you declare them. For fundamental types, you can assign a value directly:

2. Memory Leaks
The Mistake: C++ uses manual memory management, thus any memory you allocate must be explicitly released. When this is overlooked memory leaks occur, causing memory to be lost indefinitely until the application ends.

How to Avoid: To prevent memory leaks, use smart pointers like ‘std::unique_ptr’ or ‘std::shared_ptr’, which automatically manage memory and ensure it is freed when no longer needed.

When manual memory management is necessary, always pair new with delete:

3. Dangling Pointers
The Mistake: A pointer that still points to memory that has already been released is known as a dangling pointer. When you employ a dangling pointer, your software may perform erratically or crash.
Example:

How to Avoid: After deleting a pointer, immediately set it to ‘nullptr’ to avoid accidentally using it:

Dangling pointers are less problematic when utilizing smart pointers because they take care of memory deallocation automatically.
4. Off-by-One Errors
The Mistake: Loops frequently contain off-by-one errors, especially when iterating over arrays or containers. This error arises from setting the loop boundary incorrectly, either by including or excluding an index that shouldn't be there.
Example:

How to Avoid: Ensure your loop conditions correctly reflect the size of the array or container:

Such issues can also be avoided by using standard library functions like ‘std::vector::size()’, which abstract away the necessity for explicit array size management.
5. Incorrect Use of const
The Mistake: If you misuse or ignore ‘const’, it can cause changes you didn't mean to happen or stop you from making some improvements. ‘const’ is a powerful way to show purpose and make sure that certain variables or function parameters are not changed.

How to Avoid: Use ‘const’ whenever a variable or parameter should not be modified. This not only protects against accidental changes but also helps the compiler optimize your code.

You can also use ‘const’ with pointers to protect the pointed-to data:

6. Mismanagement of Object Slicing
The Mistake: When an object of a derived class is assigned to an object of a base class, the derived class's distinctive attributes are lost, a process known as object slicing. This may result in unfinished objects that exhibit unexpected behavior.
Example:

How to Avoid: To prevent object slicing, use pointers or references when dealing with polymorphism:

Alternatively, use smart pointers:

7. Overusing ‘new’ and ‘delete’
The Mistake: Manual memory management is possible in C++, but excessive use of new and delete can lead to errors and make programs more difficult to maintain. The likelihood of memory leaks, dangling pointers, and other associated problems grows with manual memory management.
Example:

How to Avoid: Give preference to standard containers (such as std::string and std::vector) or stack allocation over dynamic allocation. These minimize the requirement for new and delete data by automatically managing memory:

When dynamic allocation is necessary, prefer smart pointers:

8. Not Handling Exceptions
The Mistake: Although C++ offers a strong exception-handling system, inappropriate or non-compliant handling of exceptions can result in program crashes or abrupt terminations.
Example:
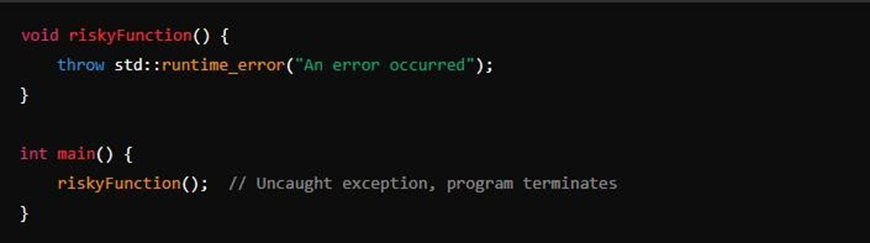
How to Avoid: Always use try-catch blocks to handle exceptions, ensuring your program can recover or at least fail gracefully:

Make sure exceptions are handled correctly in crucial code portions to avoid resource leaks or inconsistent program states.
9. Using Deprecated or Unsafe Functions
The Mistake: Some old C methods, such as ‘gets()’ and ‘strcpy()’, are inherited by C++ and are unsafe. This might result in buffer overflows or other security flaws.
Example:

How to Avoid: Always use safer alternatives provided by C++:

For string operations, prefer the ‘std::string’ class, which manages memory automatically and avoids many common pitfalls:

10. Ignoring Compiler Warnings
The Mistake:Compiler warnings are frequently signs that there might be problems with your code. Ignoring them may result in subtle flaws that are challenging to identify.
Example:

How to Avoid: Treat compiler warnings as errors during development. This can often be done by enabling specific compiler flags, like -Wall in GCC or Clang:

Address warnings promptly, and refactor your code as necessary to eliminate them. This practice helps catch potential bugs early and ensures your code is more robust.
Conclusion
C++ has a lot of features that give you fine-grained control over your programs. However, because you have so much control, you could make mistakes. You can write more reliable, efficient, and easy to maintain C++ code if you know about and avoid these typical mistakes.
Once you have built these habits, try signing up at Pro5 and seek opportunities for C++ Development!